Anyway, long story short, after checking multiple different variations of wiring and dumbing down the code to the absolute minimum, I keep getting the same result - both transceivers seem to be functioning no problem (or I'm horrifically mistaken about something), but no messages go through.
Transmitter
#include <SPI.h>
#include <nRF24L01.h>
#include <RF24.h>
RF24 radio(D1, D2); // CE, CSN
uint8_t address[][6] = {"1Node","2Node"};
void setup() {
Serial.begin(9600);
delay(1000);
if(!radio.begin())
Serial.println("init failed");
else
Serial.println("init ok");
// radio.setDataRate( RF24_2MBPS ) ;
// radio.setPALevel( RF24_PA_MAX ) ;
radio.enableDynamicPayloads() ;
radio.setAutoAck( true ) ;
radio.powerUp() ;
radio.openWritingPipe(address[1]);
radio.setPALevel(RF24_PA_MIN);
radio.stopListening();
//
// Print out the configuration of the rf unit for debugging
//
radio.printPrettyDetails();
}
void loop() {
const char text[] = "Hello World";
if(!radio.write(&text, sizeof(text)))
Serial.println("Sending failed");
delay(1000);
}
Reciever
#include <SPI.h>
#include <nRF24L01.h>
#include <RF24.h>
RF24 radio(D1, D2); // CE, CSN
uint8_t address[][6] = {"1Node","2Node"};
void setup() {
Serial.begin(9600);
delay(1000);
if(!radio.begin())
Serial.println("init failed");
else
Serial.println("init ok");
radio.enableDynamicPayloads() ;
radio.setAutoAck( true ) ;
radio.powerUp() ;
radio.begin();
radio.openReadingPipe(0, address[1]);
radio.setPALevel(RF24_PA_MIN);
radio.startListening();
radio.printPrettyDetails();
}
void loop() {
if (radio.available()) {
char text[32] = "";
radio.read(&text, sizeof(text));
Serial.println(text);
}
else
Serial.println("No message recieved");
}
Wiring for both is identical
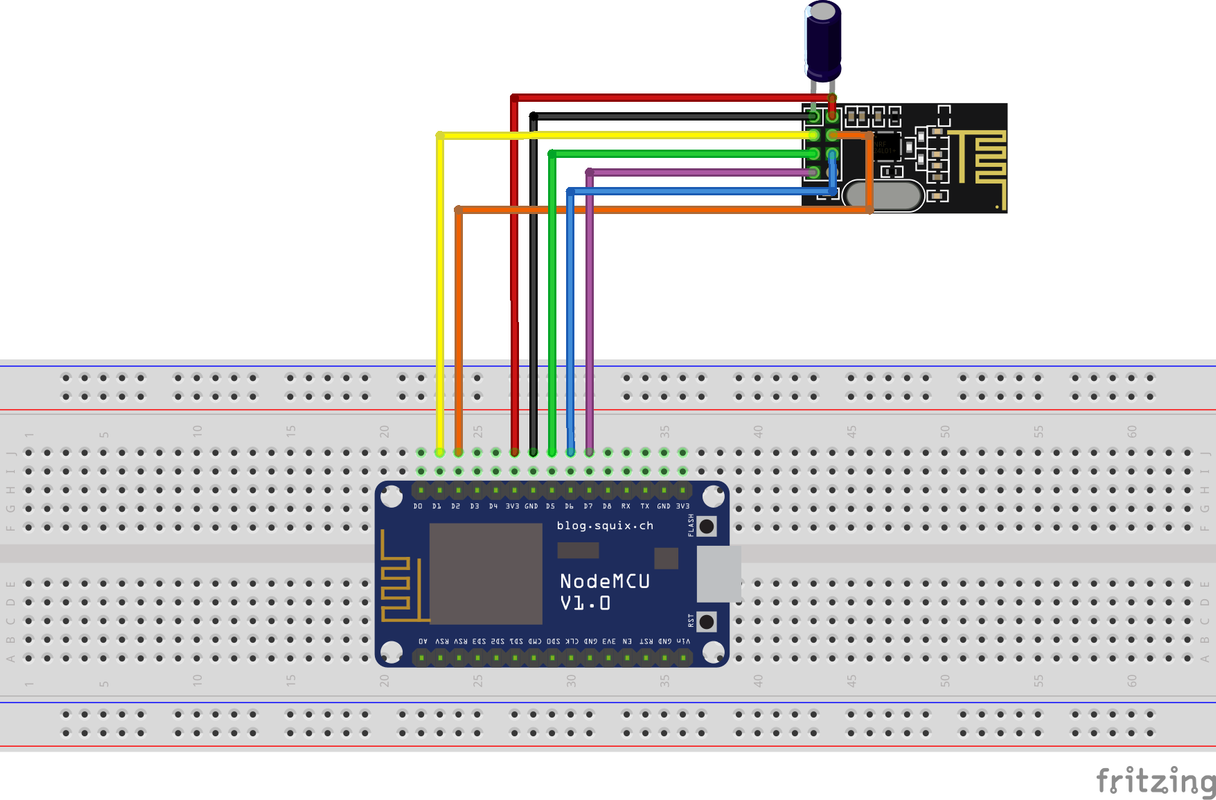
printPrettyDetails() dump
init ok
SPI Frequency = 10 Mhz
Channel = 76 (~ 2476 MHz)
RF Data Rate = 1 MBPS
RF Power Amplifier = PA_MIN
RF Low Noise Amplifier = Enabled
CRC Length = 16 bits
Address Length = 5 bytes
Static Payload Length = 32 bytes
Auto Retry Delay = 1500 microseconds
Auto Retry Attempts = 15 maximum
Packets lost on
current channel = 0
Retry attempts made for
last transmission = 15
Multicast = Disabled
Custom ACK Payload = Disabled
Dynamic Payloads = Enabled
Auto Acknowledgment = Enabled
Primary Mode = TX
TX address = 0x65646f4e32
pipe 0 ( open ) bound = 0x65646f4e32
pipe 1 ( open ) bound = 0x65646f4e32
pipe 2 (closed) bound = 0xc3
pipe 3 (closed) bound = 0xc4
pipe 4 (closed) bound = 0xc5
pipe 5 (closed) bound = 0xc6
init ok
SPI Frequency = 10 Mhz
Channel = 76 (~ 2476 MHz)
RF Data Rate = 1 MBPS
RF Power Amplifier = PA_MIN
RF Low Noise Amplifier = Enabled
CRC Length = 16 bits
Address Length = 5 bytes
Static Payload Length = 32 bytes
Auto Retry Delay = 1500 microseconds
Auto Retry Attempts = 15 maximum
Packets lost on
current channel = 0
Retry attempts made for
last transmission = 0
Multicast = Disabled
Custom ACK Payload = Disabled
Dynamic Payloads = Disabled
Auto Acknowledgment = Enabled
Primary Mode = RX
TX address = 0x65646f4e32
pipe 0 ( open ) bound = 0x65646f4e32
pipe 1 ( open ) bound = 0x65646f4e31
pipe 2 (closed) bound = 0xc3
pipe 3 (closed) bound = 0xc4
pipe 4 (closed) bound = 0xc5
pipe 5 (closed) bound = 0xc6
Sny idea what I'm missing here?