Am I doing something wrong? Code is below the schematic...
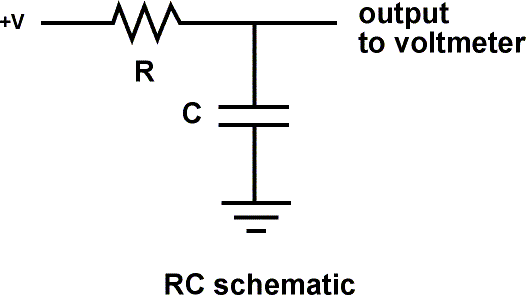
const int chargePin = 14;
const int drainPin = 12;
unsigned long chargeTime = 0;
void setup() {
pinMode(chargePin, OUTPUT);
pinMode(drainPin, INPUT);
digitalWrite(chargePin, LOW);
Serial.begin(115200);
delay(10);
Serial.println();
Serial.println();
Serial.print("Connecting to ");
Serial.println(ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
}
void loop() {
unsigned long cycleStart = 0;
// drain cap first
pinMode(drainPin, OUTPUT);
digitalWrite(drainPin, LOW);
digitalWrite(chargePin, HIGH);
Serial.print(micros()); Serial.print(" - ");
Serial.println("Discharging...");
delay(9000);
cycleStart = micros();
Serial.print(micros()); Serial.print(" - "); Serial.println("Charging!!!");
pinMode(drainPin, INPUT);
digitalWrite(chargePin, LOW);
Serial.println(digitalRead(drainPin));
while (digitalRead(drainPin) == 0) {yield();};
chargeTime = micros() - cycleStart;
Serial.print(micros()); Serial.print(" - "); Serial.print("Done...");
Serial.print(chargeTime);
Serial.println("ms");
delay(1000);
}