I'm new to the ESP8266 and working on a battery-powered ESP8266 device and having some issues with reliability of the device reporting after deep sleep. The device is set to deep sleep for 10 minutes, report the sensor reading over MQTT then go back to sleep:
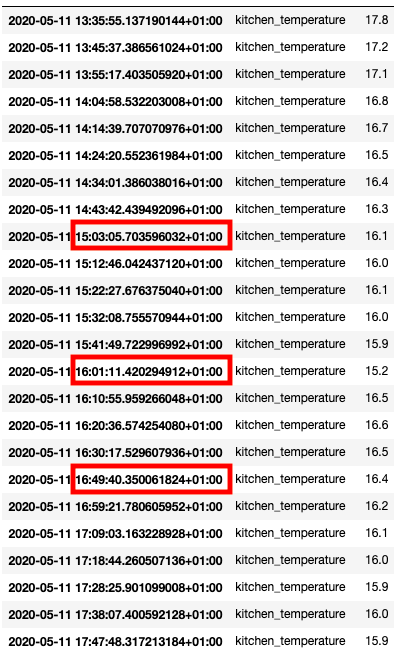
I've highlighted periods where it misses a report.
Here is the circuit:
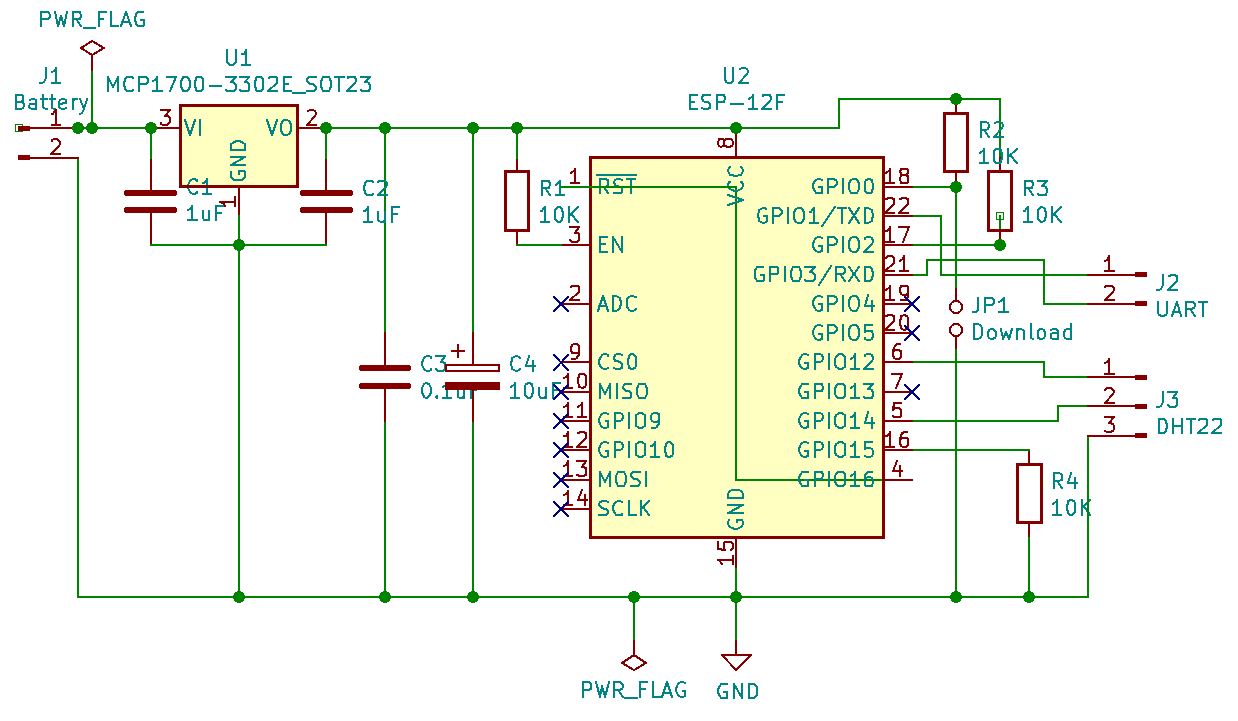
Here is the sketch:
/*
* ESP-12F with DHT22 (battery-powered)
*
* MQTT Temperature and Humidity Sensor
*
* Designed for integration with HomeAssistant
*
*/
#include <DHTesp.h>
#include <ESP8266WiFi.h>
#include <PubSubClient.h>
WiFiClient espClient;
PubSubClient client(espClient);
DHTesp dht;
// Serial debug output
#define debug false
// Device details
#define device "test" // will prefix later parameters
#define dhtDataPin 14 // GPIO14
#define dhtPowerPin 12 // GPIO12
// WiFi credentials.
#define wifi_hostname device "-sensor"
#define wifi_ssid "SSID"
#define wifi_password "PASSPHRASE"
IPAddress ip(0,0,0,0);
IPAddress gateway(0,0,0,0);
IPAddress subnet(255,255,255,0);
// Reporting
#define polling_m 10 // every X minutes
#define polling_s polling_m * 60
#define polling_ms polling_s * 1000
#define polling_us polling_ms * 1000
#define mqtt_server "IP.AD.DRE.SS"
#define humidity_topic device "/humidity"
#define temperature_topic device "/temperature"
void setup() {
// switch off WiFi until we need it
WiFi.mode(WIFI_OFF);
WiFi.forceSleepBegin();
delay(1);
// switch on the DHT22 sensor
pinMode(dhtPowerPin,OUTPUT);
digitalWrite(dhtPowerPin, HIGH);
dht.setup(dhtDataPin, DHTesp::DHT22); // Connect DHT sensor
if (debug) {
Serial.begin(115200);
while (!Serial) {}
Serial.print("Connecting to ");
Serial.println(wifi_ssid);
}
WiFi.forceSleepWake();
delay(1);
WiFi.persistent(false);
// Bring up the WiFi connection
WiFi.mode(WIFI_STA);
// Static IP assignment
WiFi.config(ip,gateway,subnet);
WiFi.hostname(wifi_hostname);
WiFi.begin(wifi_ssid, wifi_password);
while (WiFi.status() != WL_CONNECTED) {
delay(100);
if (debug) Serial.print(".");
}
float humidity = dht.getHumidity();
float temperature = dht.getTemperature();
if (debug) {
Serial.println("");
Serial.println("WiFi connected");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
Serial.println();
Serial.print("Temperature (C): ");
Serial.println(temperature);
Serial.print("Humidity (%): ");
Serial.println(humidity);
}
client.setServer(mqtt_server, 1883);
if (!client.connected()) {
if (debug) Serial.print("Attempting MQTT connection...");
if (client.connect("ESP8266Client")) {
if (debug) Serial.println("connected");
} else {
if (debug) {
Serial.print("failed, rc=");
Serial.print(client.state());
Serial.println(" trying again in 2 seconds");
}
// Wait 2 seconds before retrying
delay(2000);
}
}
client.publish(temperature_topic, String(temperature).c_str(), true);
client.publish(humidity_topic, String(humidity).c_str(), true);
client.disconnect(); // make sure the message is sent
if (debug) Serial.println("Going to sleep");
// switch off DHT22 sensor
digitalWrite(dhtPowerPin, LOW);
ESP.deepSleep(polling_us, WAKE_RF_DISABLED ); // in uS
} // end setup
void loop() {
} // end loop
So my questions are:
1. Is this expected? I have read about unreliability of the chip/WiFi in general so should I be happy with the above results?
2. I'm not pulling RST HIGH as I saw there is a 12K internal PULLUP resistor. Should I also add a 10K PULLUP?
Any other feedback on getting a long-lasting low powered device working very welcome.
Thanks,
A