I have an ESP12F with DHT22 sensor that publishes to MQTT over WiFi, then goes to deep sleep for 10 minutes, and tries again. I am using the Arduino IDE.
I've had really inconsistent behaviour where sometimes it works fine, sometimes it gets stuck in a WiFi blackhole: on boot it connects fine, but after deep sleep, it cannot find any networks at all.
I'm trying to minimise battery power so use
WiFi.mode(WIFI_OFF);
WiFi.forceSleepBegin();
delay(1);
at the start of the code, and when I know I need to use WiFi use:
WiFi.forceSleepWake();
delay(1);
WiFi.persistent(false);
// Bring up the WiFi connection
WiFi.mode(WIFI_STA);
// Static IP assignment
WiFi.config(ip,gateway,subnet);
WiFi.hostname(wifi_hostname);
WiFi.begin(wifi_ssid, wifi_password);
int count = 0;
while (WiFi.status() != WL_CONNECTED) {
if (count > 50) { // 5 seconds
if (debug) {
Serial.println("WiFi connection timeout, dropping back into deep sleep");
Serial.println(WiFi.status());
int n = WiFi.scanNetworks(false, true);
Serial.print(n);
Serial.println(" WiFi network(s) detected");
String ssid;
uint8_t encryptionType;
int32_t RSSI;
uint8_t* BSSID;
int32_t channel;
bool isHidden;
for (int i = 0; i < n; i++)
{
WiFi.getNetworkInfo(i, ssid, encryptionType, RSSI, BSSID, channel, isHidden);
Serial.printf("%d: %s, Ch:%d (%ddBm) %s %s\n", i + 1, ssid.c_str(), channel, RSSI, encryptionType == ENC_TYPE_NONE ? "open" : "", isHidden ? "hidden" : "");
}
}
ESP.deepSleep(polling_us, WAKE_RF_DISABLED ); // in uS
}
delay(100);
if (debug) Serial.print(".");
count++;
}
This is the output with serial debugging:
13:50:36.068 -> Connecting to SSID
13:50:36.172 -> fpm close 3
13:50:36.172 -> mode : sta(dc:4f:22:1d:a3:24)
13:50:36.172 -> add if0
13:50:36.279 -> ............................scandone
13:50:39.026 -> no SSID found, reconnect after 1s
13:50:39.093 -> .reconnect
13:50:39.199 -> ......................WiFi connection timeout, dropping back into deep sleep
13:50:41.293 -> 6
13:50:41.293 -> scandone
13:50:43.490 -> scandone
13:50:43.490 -> 0 WiFi network(s) detected
13:50:43.490 -> del if0
13:50:43.490 -> usl
13:50:43.557 -> enter deep sleep
The schematic is:
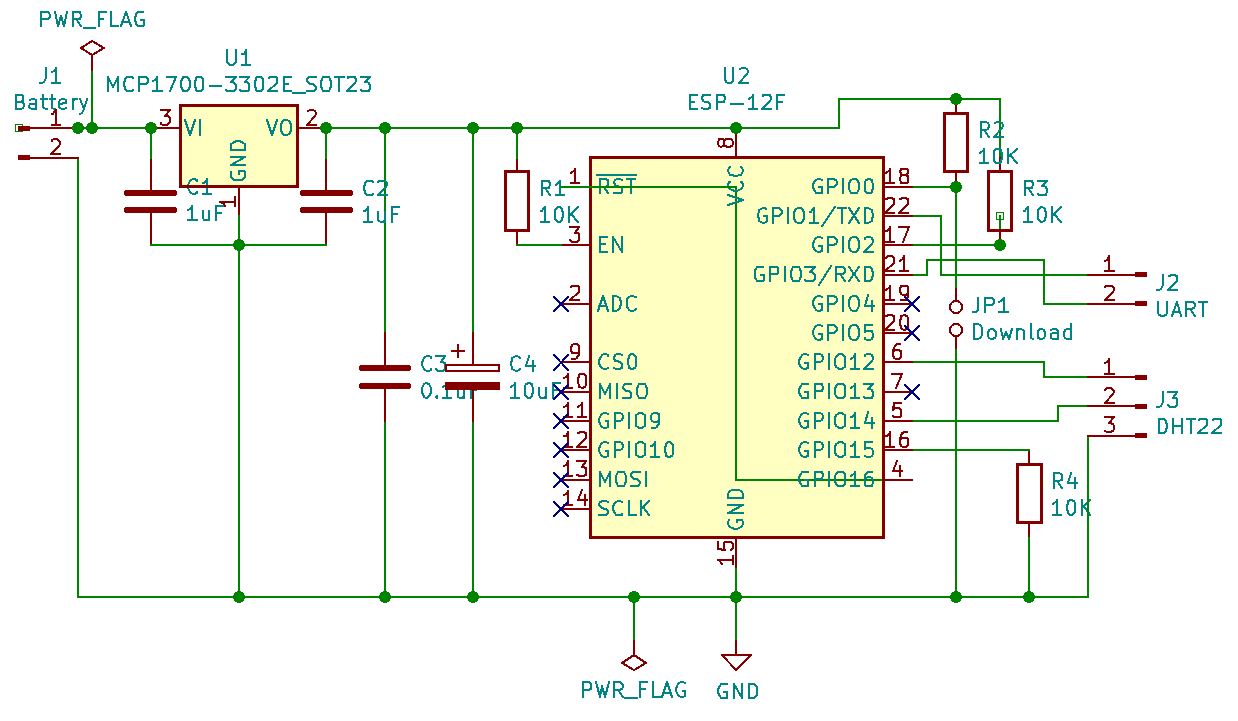
The full code is here.
Any help appreciated - I've been trying different things over the last couple of weeks and can't seem to shake this - hopefully something newbie-related that I've missed.
A